Find a number is Armstrong number or not using Python
Get a number and sum the digits of the number.
Sample Input 1:
Enter the number: 153
Sample Output 1:
Armstrong Number
Sample Input 2:
Enter the number: 213
Sample Output 2:
Not an Armstrong Number
Flow Chart Design
Try your Solution
Strongly recommended to Solve it on your own, Don't directly go to the solution given below.
Program or Solution
num = int(input("enter a number"))
n = num
count = 0
while n != 0:
count = count + 1
n = n // 10
n = num
total = 0
while n!= 0:
rem = n % 10
total = total + (rem**count)
n = n // 10
if num == total:
print("Armstrong number")
else:
print("Not an Armstrong number")
Output
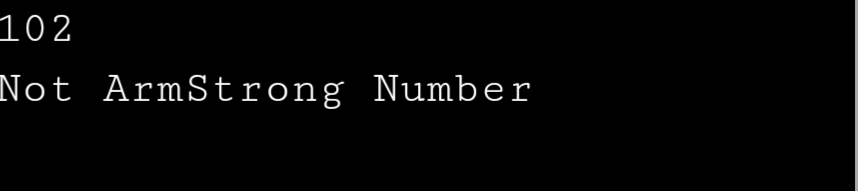
Program Explanation
using a while loop, count the number of digits in a number
initialize total is equal to 0
calculate the remainder of a number by doing number % 10, add the remainder count to the total and divide the number by the 10 and repeat the above steps till number becomes zero.
Check total is equal to original number entered by user, if it is equal print Armstrong Number else Not an Armstrong Number.
Comments
Related Programs
- Count the number of digits in a Number in Python
- Find given number is a digit in a number using Python
- Count the Number of Occurrences of digit in a number using Python
- Print the first Digit of a Number using Python
- Sum of Digits of the number using Python
- Product of Digits of the number using Python
- Reverse the digits of a number using Python