Reverse the digits of a number using Python
Get a number and reverse the digits of the number.
Sample Input 1:
Enter the number: 234
Sample Output 1:
432 is reverse of 234
Flow Chart Design
Try your Solution
Strongly recommended to Solve it on your own, Don't directly go to the solution given below.
#write your code here
Program or Solution
num = int(input("enter a number"))
n = num
reverse = 0
while n != 0:
rem = n % 10
reverse = reverse * 10 + rem
n = n // 10
print("{} is reverse of {}".format(reverse,num))
Output
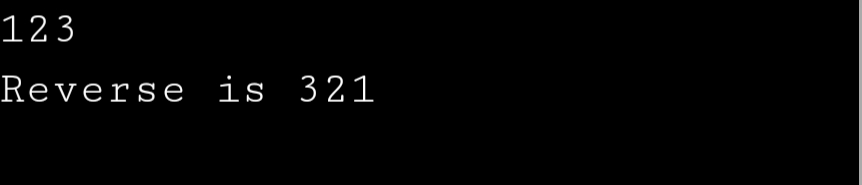
Program Explanation
initialize total is equal to 0
calculate the remainder of a number by doing number % 10, add the remainder to (total * 10) and divide the number by the 10 and repeat the above steps till number becomes zero.
Print the total, you will get the sum of digits of the number.
Comments
Related Programs
- Count the number of digits in a Number in Python
- Find given number is a digit in a number using Python
- Count the Number of Occurrences of digit in a number using Python
- Print the first Digit of a Number using Python
- Sum of Digits of the number using Python
- Product of Digits of the number using Python
- Find a number is Armstrong number or not using Python
coming Soon
coming Soon