Ternary Operator Example in Java
Ternary operator is a conditional operator which has three operands. It is best replacement one-liner for simple if-else statement.
The first operand of ternary operator is a boolean value or expression that returns boolean value. In the next two operands (or statements), either of the operand will be executed based on the value of first operand.
The below Program illustrates the working principles of ternary operator.
condition?statement1:statement2
Try your Solution
Strongly recommended to Solve it on your own, Don't directly go to the solution given below.
Program or Solution
class Ternary
{
public static void main(String args[])
{
int a = 10;
int b = 20;
int c = a>b?a:b;
System.out.println(c);
System.out.println(true?"Decode":"School");
}
}
Output
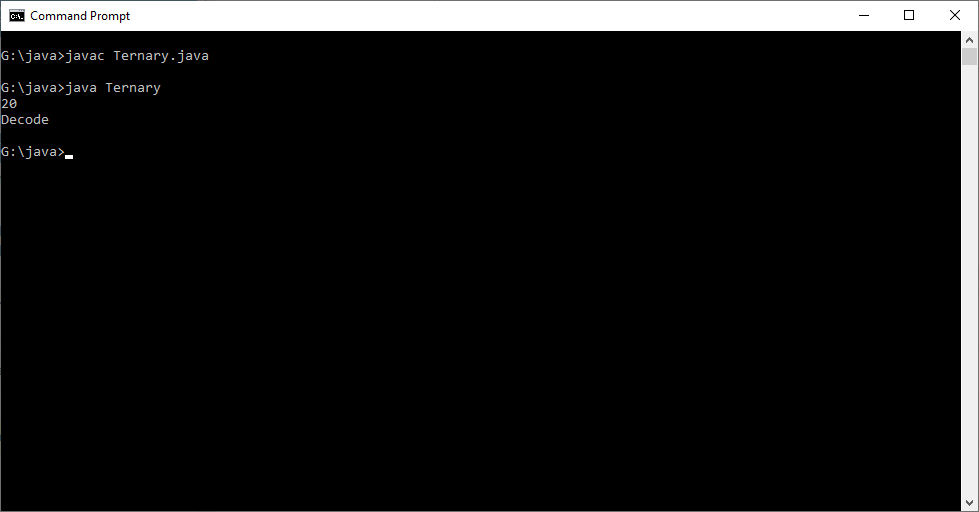
Program Explanation
In the First println() a is not greater than b so c is 20.
In the second println() first operand is true so it prints "Decode"
Comments
Related Programs
- Java Program to Addition of two numbers
- Java Program to subtraction of two numbers
- Java Program to multiply two numbers
- Java Program to divide two numbers
- Java Program to find modulus of two numbers
- Java Program to Kilo Meters to Meters
- Java Program to Meters to Kilo Meters
- Java Program to find area of Square
- Java Program to find area of Rectangle
- Java Program to find area of Right-angled triangle
- Java Program to find area of triangle
- Java Program to find area of Circle (Use Constant)
- Java Program to find the distance between two points in 2D space
- Java Program to calculate kilobytes to bytes
- Java Program to calculate bytes to kilobytes
- Java Program to find simple interest
- Java Program to calculate Fahrenheit to Celsius
- Java Program to calculate Celsius to Fahrenheit
- Java Program to Swap two numbers using third variable
- Java Program to Swap of two numbers without using third variable
- Java Program to print the last digit of given number N
- Java Program to Calculate Salary for Employees
- Post Increment Operator Example in Java
- Pre Increment Operator Example in Java
- Pre Increment and Post Increment Difference in java with Example
- Relational Operators Example in java
- Logical Operators Example in Java